Whether you’re an experienced programmer or just starting out, it’s important to understand the fundamentals of the Java language and know what questions to expect. Today we'll provide a list of common entry level Java developer interview questions with answers.
So, who is a Java Developer 👨🏼💻?
A Java developer is a software engineer who develops, tests and deploys applications written in the Java programming language. Java is an object-oriented programming language created by James Gosling and released in 1995. It is designed to be platform independent and secure, and it is one of the most popular programming languages in the world.
The List of Interview Questions 📑 for Java Developers
NOTICE: this is not a full list of interview questions, there will be at least 2-3 parts in future blog posts.
When interviewing for a position as a Java developer, it’s important to be prepared to answer questions related to the language.
Here are some common questions that you may be asked. We divided all the questions and answers into 2 columns, try to read and answer without reading the answer first - it will help you to prepare better for an interview:
- What is the difference between a constructor and a method?
- What is the difference between checked and unchecked exceptions?
- What is the purpose of garbage collection in Java?
- What is the purpose of an interface in Java?
- What are the advantages of using Java for development?
- What are the features of the Java Programming language?
- What is a ClassLoader?
- What do you get in the Java download file? How do they differ from one another?
- What are the Memory Allocations available in JavaJava?
- What are the differences between Heap and Stack Memory in Java?
- Will the program run if we write static public void main?
- What is the default value stored in Local Variables?
- What is an Association?
- What do you mean by aggregation?
- What is a Marker Interface?
- Define Copy Constructor in Java
- What is an object-oriented paradigm?
- What is Object Cloning?
- Define Wrapper Classes in Java.
- Define package in Java.
- Can you implement pointers in a Java Program?
- Explain Java String Pool.
- What is the difference between the program and the process?
- What is the final keyword in Java?
- What happens when the main() isn't declared as static?
- For more questions and answers check our another blog post here.
Answers:
- A constructor is a special method used to create and initialize objects, while a method is a block of code that performs a specific task on an object.
- Checked exceptions are those that must be handled by the programmer, while unchecked exceptions are those that are not checked at compile time and can occur at any time during execution.
- Garbage collection is an automated process that frees up memory by releasing objects that are no longer needed. This helps to improve performance and prevent memory leaks.
- An interface allows you to define a set of methods that must be implemented by any class that implements the interface. This allows for code reuse and ensures that classes are consistent with each other.
- Java offers robust performance, support for multiple platforms, scalability, security, and easy maintenance due to its object-oriented design. It also provides excellent debugging capabilities and a large library of built-in functions.
- Features:
- Easy: Java is a language that is considered easy to learn. One fundamental concept of OOP Java has a catch to understand.
- Secured Feature: Java has a secured feature that helps develop a virus-free and tamper-free system for the users.
- OOP: OOP stands for Object-Oriented Programming language. OOP signifies that, in Java, everything is considered an object.
- Independent Platform: Java is not compiled into a platform-specific machine; instead, it is compiled into platform-independent bytecode. This code is interpreted by the Virtual Machine on which the platform runs. - A classloader in Java is a subsystem of Java Virtual Machine, dedicated to loading class files when a program is executed; ClassLoader is the first to load the executable file. Java has Bootstrap, Extension, and Application classloaders.
- JDK - Java Development Kit (JDK is a dedicated kit for solely software development, platform dependent, contains of a set of tools for debugging and developing, represented as an installer file);
JRE - Java Runtime Environment (a set of software and library designed for executing Java Programs, also platform dependent, its package is one that only supports files and libraries for a runtime environment, represented as runtime environment, not an installer). - There are 5:
- Class Memory
- Heap Memory
- Stack Memory
- Program Counter-Memory
- Native Method Stack Memory - Stack memory is the amount of memory allocated to each individual program. It is a fixed memory space. Heap memory, in contrast, is the portion that was not assigned to the Java code but will be available for use by the Java code when it is required, which is generally during the program's runtime.
- Yes, the program will successfully execute if written so. Because, in Java, there is no specific rule for the order of specifiers
- Neither the Local Variables nor any primitives and Object references have any default value stored in them.
- An Association can be defined as a relationship that has no ownership over another. For example, a person can be associated with multiple banks, and a bank can be related to various people, but no one can own the other.
- The term aggregation refers to the relationship between two classes best described as a “whole/part” and “has-a” relationship. This kind is the most specialized version of an association relationship. It contains the reference to another class and is said to have ownership of that class.
- An empty interface in Java is referred to as a Marker interface. Serializable and Cloneable are some famous examples of Marker Interface.
- A Copy Constructor in Java is a constructor that initializes an object through another object of the same class.
- A Paradigm that is based on the concepts of “Objects.” It contains data and code. Data that is in the form of fields, and regulation, that is in the form of procedures. The exciting feature of this paradigm is that the object’s procedures can access and often modify the data fields themselves.
- An ability to recreate an object entirely similar to an existing object is known as Object Cloning in Java. Java provides a clone() method to clone a current object offering the same functionality as the original object.
- In Java, when you declare primitive datatypes, then Wrapper classes are responsible for converting them into objects(Reference types).
- The package is a collective bundle of classes and interfaces and the necessary libraries and JAR files. The use of packages helps in code reusability.
- Accessing memory directly through pointers is not a recommended action. Hence, pointers are eliminated in Java.
- A collection of strings in Java's Heap memory is referred to as Java String Pool. In case you try to create a new string object, JVM first checks for the presence of the object in the pool. If available, the same object reference is shared with the variable, else a new object is created.
- A programme is a non-active entity that includes the collection of codes necessary to carry out a specific operation. When a programme is run, an active instance of the programme called a process is launched. A process is begun by a programme once it has been run. The process carries out the program's specified instructions.
- The term final is a predefined word in Java that is used while declaring values to variables. When a value is declared using the final keyword, then the variable's value remains constant throughout the program's execution.
- When the main method is not declared as static, then the program may be compiled correctly but ends up with a severe ambiguity and throws a run time error that reads "NoSuchMethodError."
- For more questions and answers check another blog article here.
Understanding your responsibilities 🪚
Understanding what's waiting for you when you will start working is also an important thing and showing it on your job interview will increase your chances of landing a dream job.
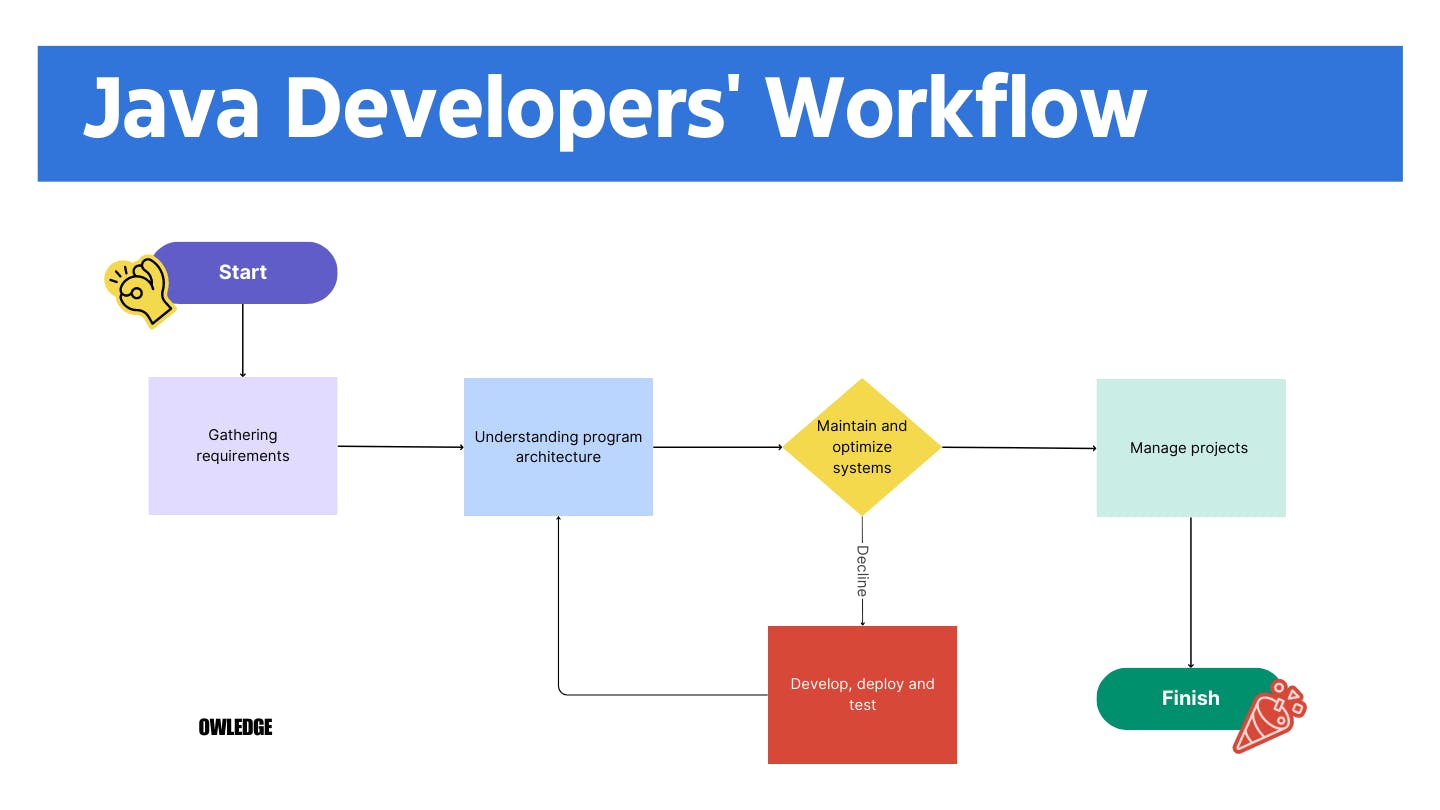
But you need to understand that everything depends on company you will be working on, smaller company = more responsibilities:
- Gathering requirements: gathering requirements from the client will help you and your team to build a process of creating web, app or whatever you want to build;
- Understanding program architecture: understanding the overall vision of what needs to be accomplished and need the skills of a Java Developer to complete their project;
- Maintain and optimize systems: providing software updates, test code, implementing new features, preparing End User Documentation, maintaining projects, providing code optimization, identifying other programs that could enhance current systems;
- Develop, Test and Deploy code;
- Manage projects: more for experienced developers, team leaders, senior developers;
- Vendor management: conceptualizing Vendor Management strategy, preparing an RFQ questionnaire and other bid documents, evaluating offers and negotiate with vendors, selecting vendors and maintain relationships, implementing a strategy for vendor accountability.
Conclusion 🔗
Answering interview questions, understanding your responsibilities and company's workflow + being confident enough on interview = happy you, happy customers and good salary of course as a reward. With these things in mind, you will be able to demonstrate your knowledge and land your dream job!
Next batch of interview questions you can find here.
### Useful Links
What is Owledge? - Learn more about Owledge in 1 short article.
Join Our Discord Community - we post 100+ freelancing jobs, news and trends daily!
Try Owledge For Free - to explore our app for freelancers, agencies, solopreneurs and small businesses!
You can also follow us on Facebook, Twitter, Pinterest & LinkedIn.