This is a second part of our blog where we discuss even more common entry level Java developer interview questions that will definitely help you to learn more. We’ll also provide tips on how to stay confident and focused during the interview process.
The List of Entry Level Interview Questions 📑 for Java Developers
NOTICE: this is a part 2 of interview questions. part 1 is available here.
When interviewing for a position as a Java developer, it’s important to be prepared to answer questions related to the language (read the question first and try to answer without scrolling down to answers). Here are some common questions that you may be asked:
- Why is the main method static in Java?
- What happens if the static modifier is not included in the main method signature in Java?
- Can we make the main() thread a daemon thread?
- What happens if there are multiple main methods inside one class in Java?
- How does an exception propagate in the code?
- Is it mandatory for a catch block to be followed after a try block?
- Can you call a constructor of a class inside another constructor?
- Why does the java array index start with 0?
- Why is the remove method faster in the linked list than in an array?
- How does the size of ArrayList grow dynamically? And also state how it is implemented internally?
- What are Composition and Aggregation? State the difference.
- How is the creation of a String using new() different from that of a literal?
- How is the ‘new' operator different from the ‘newInstance()' operator in java?
- Is exceeding the memory limit possible in a program despite having a garbage collector?
- Why is synchronization necessary? Explain with the help of a relevant example.
- Define System.out.println().
- Can you explain the Java thread lifecycle?
- Is it possible to import the same class or package twice in Java and what happens to it during runtime?
- In case a package has sub packages, will it suffice to import only the main package? e.g. Does importing of com.myMainPackage.* also import com.myMainPackage.mySubPackage.*?
- Will the final block be executed if the code System.exit(0) is written at the end of the try block?
- Explain the term “Double Brace Initialisation” in Java?
- What are the possible ways of making objects eligible for garbage collection (GC) in Java?
- What is the best way to inject dependency? Also, state the reason.
- How we can set the spring bean scope. And what supported scopes does it have?
- What are the different categories of Java Design patterns?
- What is a Memory Leak? Discuss some common causes of it.
- Is delete, next, main, exit or null keyword in java?
- What is a JIT compiler?
- How many types of constructors are used in Java?
- Explain ‘this’ keyword in Java.
- Explain ‘super’ keyword in Java.
- Explain Method Overloading in Java.
- Can we overload a static method?
- Define Late Binding.
Answers:
- Java's main() function is static by default, allowing the compiler to call it either before or after creating a class object. The main () function is where the compiler begins programme execution in every Java programme. Thus, the main () method needs to be called by the compiler. If the main () method is permitted to be non-static, the JVM must instantiate its class when calling the function.
- The main function is called by the JVM even before the objects are created, thus even if the code correctly compiles, there will still be an error at runtime.
- This technique designates whether the active thread is a user thread or a daemon thread. For instance, tU.setDaemon(true) would convert a user thread named tU into a daemon thread. On the other side, executing tD.setDaemon(false) would convert a Daemon thread, tD, into a user thread.
- There is no limit to the number of major approaches you can use. Overloading is the ability to have main methods with different signatures than main (String []), and the JVM will disregard those main methods.
- In the event that an exception is not caught, it is initially thrown from the top of the stack and then moves down the call stack to the preceding method. The runtime system looks for a way to handle an exception that a method throws. The ordered list of methods that were called to get to the method where the error occurred is the collection of potential "somethings" that can be used to manage the exception. The call stack is the list of methods, and exception propagation is the search technique.
- Each attempt block does not necessarily have to be followed by a catch block. Either a catch block or a final block ought to come after it. Additionally, any exceptions that are expected to be thrown should be mentioned in the method's throws clause.
- Yes, a class may include any number of constructors, and each function Object () {[native code] } may call the others using the this() function Object() { [native code] } call function [please do not mix the this() function Object() { [native code] } call function with this keyword]. The constructor's first line should be either this () or this(args). Overloading of constructors is what this is called.
- The distance from the array's beginning is just an offset. There is no distance because the first element is at the beginning of the array. Consequently, the offset is 0.
- Because there is no background scaling of an array, insertion, addition, and removal operations are quicker with a LinkedList. Only references in adjacent items need to update when a new item is added in the middle of the list.
- A resizable array implementation in Java is called ArrayList. Dynamically expanding array lists make it possible to add new elements at any time. The underlying data structure of the ArrayList is an array of the Object class. The ArrayList class in Java has three constructors. There are available readObject and writeObject methods specific to it. The Object Array in an ArrayList is temporary. There are implemented and Serialization-capable versions of RandomAccess, Cloneable, and java.io (that are Marker Interface in Java).
- Aggregation (HAS-A) and composition are its two forms (Belongs-to). In contrast to composition, which has a significant correlation, the aggregation has a very modest association. Aggregation can be thought of as a more confined version of the composition. Since all compositions are aggregates but not all aggregates are compositions, aggregate can be thought of as the superset of composition.
- The new () operator always produces a new object in heap memory when creating a String object. The String pool may return an existing object if we build an object using the String literal syntax, such as "Baeldung," on the other hand.
- Both the new operator and the newInstance() method are used to create objects in Java. If we already know the kind of object to create, we can use the new operator; however, if the type of object to create is supplied to us at runtime, we must use the newInstance() function.
- Yes, even with a garbage collector in place, the programme could still run out of memory. Garbage collection aids in identifying and removing programme objects that are no longer needed in order to release the resources they use. When an object in a programme cannot be reached, trash collection is executed with respect to that object. If there is not enough memory available to create new objects, a garbage collector is used to free up memory for things that have been removed from the scope. When the amount of memory released is insufficient for the creation of new objects, the program's memory limit is exceeded.
- Multiple threads trying to access the same resources in a multi-threaded software may frequently result in unexpected and incorrect outcomes. Therefore, it must be ensured through some form of synchronization that only one thread can access the resource at any given time. Java offers a method for setting up threads and synchronizing their operations with the aid of synchronized blocks. The synchronized keyword in Java is used to identify synchronized blocks. In Java, a synchronized block is one that is tied to an object. Only one thread can be running at a time inside synchronized blocks since they are all synchronized on the same object. Until the thread inside the synchronized block exits the block, all other threads trying to enter the block are blocked.
- System.out.println() in Java outputs the argument that was supplied to it. On the monitor, the println() method displays the findings. An objectname is typically used to call a method.
- These are the states:
- New: A new thread is always in the new state when it is first formed. The function hasn't been run yet, thus it hasn't started to execute for a thread in the new state.
- Active: A thread switches from the new state to the active state when it calls the start() method. The runnable state and the running state are both contained within the active state.
- Blocked or Waiting: A thread is either in the blocked state or the waiting state when it is inactive for a while (but not indefinitely).
- Timed waiting: When we use the sleep () method on a particular thread, we are actually engaging in timed waiting. The thread enters the timed wait state using the sleep () function. The thread awakens when the allotted time has passed and resumes execution where it left off.
- Termination: A thread that has been terminated means it is no longer active in the system. In other words, the thread is inactive and cannot be revived (made active again after being killed). - The same package or class may be imported more than once. Neither the JVM nor the compiler raise an objection. Even if you import the same class several times, the JVM will only internally load it once.
- Sub-packages won't be imported when a package is imported. When you import a package, all of its classes and interfaces—with the exception of those from its sub-packages—are imported.
- The system is established as the last line to be run, after which nothing will happen, therefore both the catch and finally blocks are essentially ignored.
- The outer braces of the double-brace initialization construct an anonymous class that is descended from the provided class and gives an initializer block for that class (the inner braces).
- If a reference variable for an object is removed from the programme while it is running, the object may be trash collected. They are also referred to as inaccessible objects occasionally. The new operator returns a reference to an object after dynamically allocating memory for it.
- Constructor injection. A class requesting its dependencies through its function Object() { [native code] } is the most typical instance of dependency injection. Since the client cannot be constructed without the required dependencies, this guarantees that it is always in a correct state.
- There are four ways to set the scope of a Spring bean: singleton, prototype, request, and session.
- The singleton scope creates a single instance of a bean, which is shared by all objects that request it.
- The prototype scope creates a new instance of a bean for each object that requests it.
- The request and session scopes are only available in a web-based context. The request scope creates a new bean instance for each HTTP request, and the session scope creates a single instance of a bean shared by all objects in a single HTTP session. - The three categories of Java design patterns are creational, structural, and behavioural design patterns.
- A memory leak is the slow degradation of system performance over time brought on by the fragmentation of a computer's RAM as a result of shoddy application design or programming that fails to release memory chunks when they are no longer required. These memory leaks frequently result from session items in excess, insertion into Collection objects without deletion, infinite caches, excessive page switching on the operating system, listener methods that are not called, and bespoke data structures that are poorly written.
- No, these keywords do not exist in Java. Delete, Next, Exit are the operations performed in the Java program, Main is the predefined method, and Null is the default String type.
- JIT compiler refers to Just in Time compiler. It is the simplest way of executing the computer code that takes in compilation during the execution of a program rather than before performance. It commonly uses bytecode translation to machine code. It is then executed directly.
- There are two types of constructors in Java:
- Parameterized Constructors: Parameterized constructor accepts the parameters with which users can initialize the instance variables. Users can initialize the class variables dynamically at the time of instantiating the class.
- Default constructors: This type doesn’t accept any parameters; rather, it instantiates the class variables with their default values. It is used mainly for object creation. - The term "this" is a particular keyword designated as a reference keyword. The "this" keyword is used to refer to the current class properties like method, instance, variable, and constructors.
- The term "super" is a particular keyword designated as a reference keyword. The "super" keyword refers to the immediate parent class object.
- The process of creating multiple method signatures using one method name is called Method Overloading in Java. Two ways to achieve method overloading are:
undefinedundefined - No, Java does not support the Overloading of a static method. The process would throw an error reading "static method cannot be referenced."
- Binding is a process of unifying the method call with the method's code segment. Late binding happens when the method's code segment is unknown until it is called during the runtime.
How to Stay Confident 🧑🏼💼 During an Interview
Interviews can be intimidating, but they don’t have to be! Here are some tips from for staying calm and composed during difficult questions and make sure you understand what is being asked before responding. If you don’t know the answer, don’t be afraid to say so; try to explain your thought process or suggest alternative solutions instead.
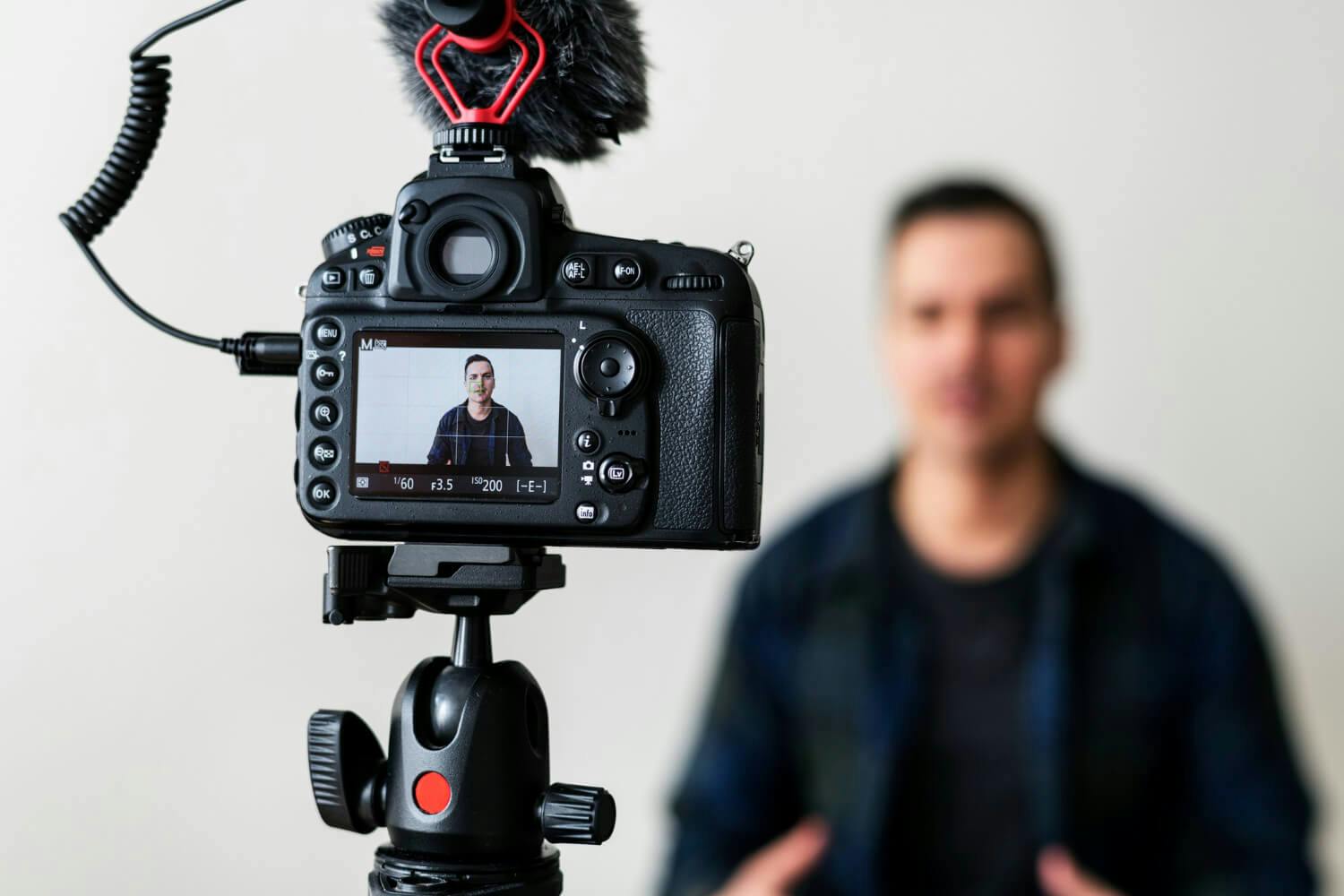
- Take deep breaths and remember that you know more than you think you do. Be prepared by researching the company and practice answering common questions beforehand.
- Visualize a successful interview and try to focus on the positives. Remember that your interviewer wants you to succeed, so don’t be afraid to ask questions if you need clarification.
- Take your time when answering difficult questions and make sure you understand what is being asked before responding. If you don’t know the answer, don’t be afraid to say so; try to explain your thought process or suggest alternative solutions instead.
- Talk about your experience with relevant projects or technologies, as well as any certifications or qualifications you have acquired. Be proactive in asking questions about the company’s needs and showing how your skills can address them.
Conclusion 🔗
Being confident and prepared are essential when attending a Java developer interview. To do this, you should research the company ahead of time, practice answering common questions, take your time when responding to difficult questions, and demonstrate your knowledge by talking about relevant experience and qualifications.
A successful interview requires confidence, preparation, and enthusiasm. With these tips/answers in mind, you will be able to demonstrate your knowledge and stand out from other candidates!
Previous batch of interview questions you can find here.
### Useful Links
What is Owledge? - Learn more about Owledge in 1 short article.
Join Our Discord Community - we post 100+ freelancing jobs, news and trends daily!
Try Owledge For Free - to explore our app for freelancers, agencies, solopreneurs and small businesses!
You can also follow us on Facebook, Twitter, Pinterest & LinkedIn.